React props
In React, we use props to make a parent component "talk" to a child component:
- the parent component will use a special kind of attribute or property known as
props
- this consists of an attribute in a JSX tag
- the attribute can take on any key name with any value
- the child component function will receive these
props
as arguments corresponding to its parameters- one
props
object will have property-value pairs corresponding to attributes - the child component's
return
function can then useprops
like a variable
- one
In App.jsx, we would introduce a someData
attribute in props
:
/* ourcraft/src/App.jsx */
import { Component } from './Component'
export default function App() {
return (
<div>
<h1>Hello!</h1>
<h2>Let's begin our React App!</h2>
<Component someData="hello component" />
</div>
)
}
Then, in Component.jsx we would bring the props
in as an argument:
/* ourcraft/src/Component.jsx */
export function Component(props) {
return (
<div>
<h3>{props.someData}</h3>
<p>A child component!</p>
</div>
)
}
To access someData
we would simply use props.someData
as shown above!
Alternate syntax
We could make things more concise in the component (let's make a separate ComponentAlternate.jsx file for clarity's sake):
/* ourcraft/src/ComponentAlternative.jsx */
export function Component({someData}) {
return (
<div>
<h3>{someData}</h3>
<p>A child component!</p>
</div>
)
}
Notice that:
- the
{someData}
parameter has curly braces, whileprops
would not- here we have engaged in JavaScript object destructuring
Moving this over to App.jsx:
/* ourcraft/src/App.jsx */
import { Component } from './Component.js'
import { ComponentAlternate } from './ComponentAlternate.js'
export default function App() {
return (
<div>
<h1>Hello!</h1>
<h2>Let's begin our React App!</h2>
<Component someData="hello component" />
<ComponentAlternate someData="alternate component" />
</div>
)
}
The output for Component
and ComponentAlternate
will look essentially identical (except for the value passed into the someData
prop):
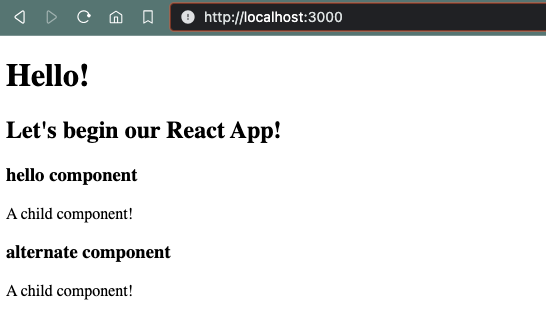
Passing in more than one parameter
We could also pass in another parameter (let's use our ComponentAlternate.jsx file):
/* ourcraft/src/ComponentAlternate.jsx */
export function ComponentAlternate({someData, otherData}) {
return (
<div>
<h3>{someData} #{otherData}</h3>
<p>A child component!</p>
</div>
)
}
Then, in the App.jsx file:
import { Component } from './Component.js'
import { ComponentAlternate } from './ComponentAlternate.js'
export default function App() {
return (
<div>
<h1>Hello!</h1>
<h2>Let's begin our React App!</h2>
<Component someData="hello component" />
<ComponentAlternate
someData="alternate component"
otherData={100}
/>
</div>
)
}
Note how we simply added an extra JSX attribute/prop called otherData
in the ComponentAlternate
tag!
The output would look like this:
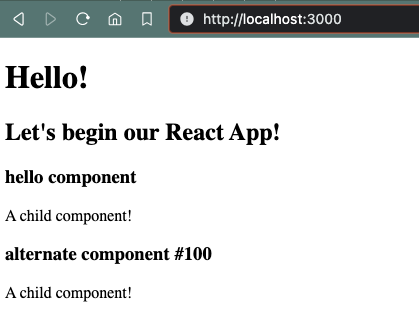